Unity
Overview
The following should be true prior to calling Configure()
on the TapResearchSDK:
- You should have your callback methods implemented.
- You should have the User Identifier set.
Now you can call Configure()
on the TapResearchSDK. This will initialize the SDK and check for any unredeemed rewards.
Step 1: Setup
- Create an app and grab your API Token.
- Add a test device
Supported versions
TapResearch only supports Unity v2017.4.0f1 and above.
Download the SDK
You can download the latest version of the TapResearch Unity SDK on GitHub. If you require a specific version you can download the SDK by tag on Github.
Integrate .unitypackage
Inside the TapResearchSDK directory, you will find TapresearchSDK.unitypackage. You can import the package by selecting Assets > Import Package > Custom Package... through the Unity menu.
External Dependency Manager
The SDK uses External Dependency Manager for Unity to integrate the native library dependencies.
If the dependency manager is already installed on your app, you can remove the Dependency Manager files when importing the SDK.
For the Android build, make sure the dependencies have been resolved by going to Assets
=> External Dependency Manager
=> Android Resolver
=> Resolve
.
For iOS, the plugin will add CocoaPods to the project and will import the dependencies in the Xcode project.
iOS Project Setup
- Ensure you have the following inside the Assets > Plugins > iOS folder:
- TapResearch.framework
- TRUnityBridge.mm
Frameworks
The TapResearchSDK includes a post-process script that runs after compilation to add required frameworks and linker flags for iOS. If you're using a Windows machine to generate the Xcode project or if the script fails, you'll need to add the frameworks and set the linker flags manually.
- Add the following frameworks to Target > Build Phases > Link Binary With Libraries:
- Foundation.framework
- UIKit.framework
- SystemConfiguration.framework
- MobileCoreServices.framework
- AdSupport.framework
- Security.framework
Linker Flags
- Select the build settings tab and type in Other Linker Flags in the search field.
- Add the following flag.
- -ObjC
Android Project Setup
If your app's targetSdk is 35 or higher, you will need to use Unity version 2.5.20 or higher. Android 15 introduced a change that will cut off the top title bar of the Survey Wall.
- Ensure you have the following inside the Assets > Plugins > Android folder:
- com.tapr.tapresearch-2.5.+.aar
- com.tapr.tapresearch-2.5.+.aar
Step 2: Callbacks
Placement Delegate
A new delegate has been added which is used by the SDK to let the app know a placement is ready or not available.
You no longer need to initialize a placememt manually. When implementing the delegate, these callbacks will alert you of a new placement for you to store its status. Placements now call showSurveyWall() or displayEvent() with a new method overload.
TapResearchSDK.OnPlacementUnavailable = this.OnPlacementUnavailable;
TapResearchSDK.OnPlacementReady = this.OnPlacementReady;
When implementing the delegate, you callbacks will alert you of a new placement for you to store its status. Placements are now passed at the time of showSurveyWall() with a new method overload.
void Awake()
{
public TRPlacement placement;
placement.ShowSurveyWall();
}
Reward Callbacks
The SDK will check if the user has unredeemed rewards in the following events:
- On SDK initialization
- When the user exits TapResearch
The RewardListener
interface is the reward listener that will handle the new rewards that the player earned in a session. The object that implements the interface should be passed to TapResearchSDK.getInstance().setRewardListener
to get activated.
The reward information will be encapsulated in the TRReward
object with the following methods:
Method name | Type | Description |
---|---|---|
TransactionIdentifier | String | The reward unique identifier |
CurrencyName | String | The virtual currency name |
PlacementIdentifier | String | The placement that started the session identifier |
RewardAmount | int | The reward amount in virtual currency. The value will automatically be converted to your virtual currency based on the exchange rate you specified in the app settings. |
PayoutEvent | int | The action that the user was rewarded for. 0 - Profile Complete, 3 - Survey Complete. |
public class MyMainController : MonoBehaviour {
public void Awake() {
TapResearchSDK.Configure (YOUR_API_TOKEN);
TapResearchSDK.OnReceiveReward = this.OnReceiveReward;
}
private void OnReceiveReward(TRReward reward) {
Debug.Log ("You've earned " + reward.RewardAmount + " " + reward.CurrencyName + ". " + reward.TransactionIdentifier);
}
}
It is important to note that onReceiveReward
will be called back-to-back if the player completed multiple surveys in one session.
Survey callback (Optional)
Assign a delegate if you want to be notified when the survey wall status changes.
public class MyMainController : MonoBehaviour {
public void Start() {
TapResearchSDK.OnSurveyWallOpened = this.OnSurveyWallOpened;
TapResearchSDK.OnSurveyWallDismissed = this.OnSurveyWallDismissed;
}
private void OnSurveyWallOpened () {
// Stop music, timers, etc.
}
private void OnSurveyWallDismissed () {
// Start music, timer, etc.
}
}
Step 3: Initialize
Initialize the TapResearchSDK as early as possible. Please note that the Configure()
method only needs to be called once on app launch.
Also, your iOS and Android apps have different API tokens. Use pre-processor directives so Unity knows which API token to use when you build your app.
using TapResearch;
#if UNITY_IOS
const String ApiToken = "ios_api_token";
#elif UNITY_ANDROID
const String ApiToken = "android_api_token";
#endif
public class MyMainController : MonoBehaviour {
public void Awake()
{
TapResearchSDK.Configure(ApiToken);
}
}
Step 4: Set User Identifier
The next step will be to set a unique user identifier. Please note that without a unique identifier, the survey wall won't be available.
TapResearchSDK.SetUniqueUserIdentifier("<UNIQUE_USER_IDENTIFIER>");
Our system only accepts User IDs with ASCII characters. If necessary, you can convert it to BASE64 before sending the User ID to us.
Step 5: Call Placements
A Placement
is an object that should be attached to the app's call to action that will direct the users to TapResearch.
To view the available placements or creating a new one, navigate to the app settings in the Supplier Dashboard, and copy the placement's identifier.
The Placement
is encapsulated in the TRPlacement
object which contains metadata and the method to display the survey wall.
An Event
is an object that should be attached to the TRPlacement
that will prompt the users with a call to action if you have created one in your supplier dashboard for that specific placement. More on event setup can be found here: Sales & Events.
public TRPlacement tapresearchPlacement;
void Start()
{
TapResearchSDK.OnPlacementReady = this.OnPlacementReady;
}
void OnPlacementEventReady(TRPlacement placement)
{
//You can now store this placement to be shown with your Survey Wall.
}
void OnPlacementUnavailable(string expiredPlacement)
{
Debug.Log("Placement expired or refreshing: " + expiredPlacement);
}
More About Placements
The survey wall may or may not be available to a specific user and it's important to check survey availability before displaying the call to action.
A placement can only show the survey wall one time. Once the survey wall is dismissed, you'll have to initialize a new
TRPlacement
object if you wish the user to go back to TapResearch.
Display the survey wall
To display the survey wall, call the ShowSurveyWall
on your TRPlacement
object.
myPlacement.ShowSurveyWall();
Displaying an Event (New!)
When placementReady
has been called then you can call displayEvent
on the placement you desire:
if (mainPlacement.IsEventAvailable()) {
mainPlacement.DisplayEvent();
}
else {
mainPlacement.ShowSurveyWall(); //SurveyWall is still available if the placement is without an event.
}
To listen to the event Open and Dismissed actions, add the following callbacks.
TapResearchSDK.OnEventOpened = this.OnEventModalOpened;
TapResearchSDK.OnEventDismissed = this.OnEventModalDismissed;
void OnEventModalOpened(TRPlacement placement)
{
Debug.Log("Event Modal Opened");
}
void OnEventModalDismissed(TRPlacement placement)
{
Debug.Log("Event Modal Dismissed");
}
Server to server callbacks
You can read more about server to server callbacks.
(Debug) Troubleshooting
Test Devices:
Before you are ready to go live, the SDK can only work with a test device. Navigate to your dashboard and click the Add Devices button. Add a device name and a unique user identifier or a Google Advertising ID / Apple IDFA. Now, when you enter our survey flow through your app, you will be able to complete a test survey and receive a test reward when you re-open your app.
Survey wall isn't available:
If placement.IsSurveyWallAvailable
is false, please reference the Android or iOS integration guides for further steps.
Android Debug Console Output:
To output debug info to logcat when generating an Android build, use the following:
#if UNITY_ANDROID
TapResearchSDK.SetDebugMode (true);
#endif
Screen Orientation for Landscape Applications
TapResearch works better in portrait mode. Using Unity Player Settings to disable Portrait orientation may result in problems rendering some surveys.
Recommended Unity Player Settings
We recommend always allowing Portrait in addition to any other needed orientations.
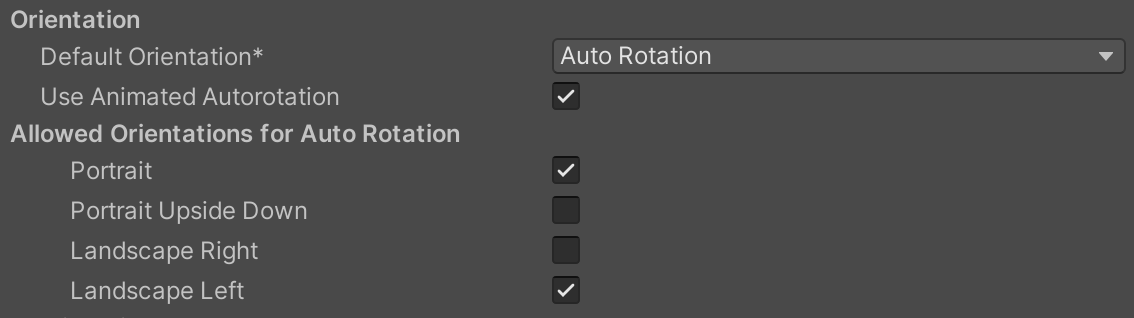
Setting an orientation using Screen.orientation
in C# scripts forces that orientation and the TapResearch SDK will show in that orientation.
Suggested Xcode Settings for iPhone and iPad
Setting orientations using the Unity Player Settings dialog sets the same values in the generated Xcode project for iOS. It sets the same values for iPhone and iPad. The TapResearch SDK works best in Portrait on iPhones, on iPad all orientations work equally well.
The example Unity Player Settings above generates the following Xcode settings:
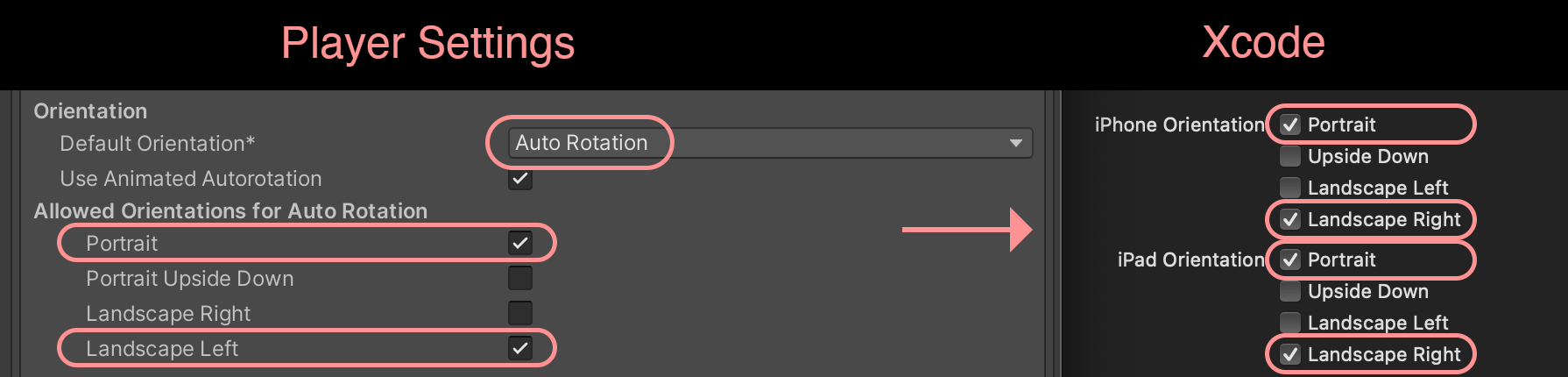
TapResearch Surveys
To make sure that TapResearch surveys display in portrait mode when you have locked your game in landscape mode set the Screen.orientation
to ScreenOrientation.Portrait
. Use co-routines or scene-loads to make sure it is in effect before opening any TapResearch content.
We recommend switching into portrait orientation if you have locked your game to landscape.
TapResearch Banners & Interstitials
Banners and interstitials can work in any orientation, however when tapped they will go on to show surveys.
For placements starting with a banner or interstitial we recommend switching to Screen.orientation
to ScreenOrientation.AutoRotation
with landscape left, right and portrait enabled for auto-rotation:
Screen.autorotateToPortrait = true;
Screen.autorotateToLandscapeLeft = true;
Screen.autorotateToLandscapeRight = true;
Unity example project
An example project for Unity can be found in GitHub. This example shows how to integrate the TapResearchSDK package and how orientation can be managed.
Unity example project orientation handling
We have included a simple orientation changer class TROrientationChanger.cs
in the example project that illustrates how to change orientation.
When our example starts we set (lock) to landscape orientation using
Screen.orientation = ScreenOrientation.LandscapeLeft;
When we are ready to show TapResearch content we use the included example orientation changer to change to portrait orientation:
orientationChanger.SetPortrait(OnOrientationChangedToPortrait);
In the completion handler for SetPortrait we show the placement:
private void OnOrientationChangedToPortrait()
{
mainPlacement.ShowSurveyWall();
}
When the user dismisses the surveys we do the reverse to return to landscape orientation:
orientationChanger.SetLandscapeLeft(OnOrientationChangedToLandscapeLeft);
Done: Going Live
Learn how to take the app live.
Contact
Please send all questions, concerns, or bug reports to developers@tapresearch.com